How to read N integers into a vector?
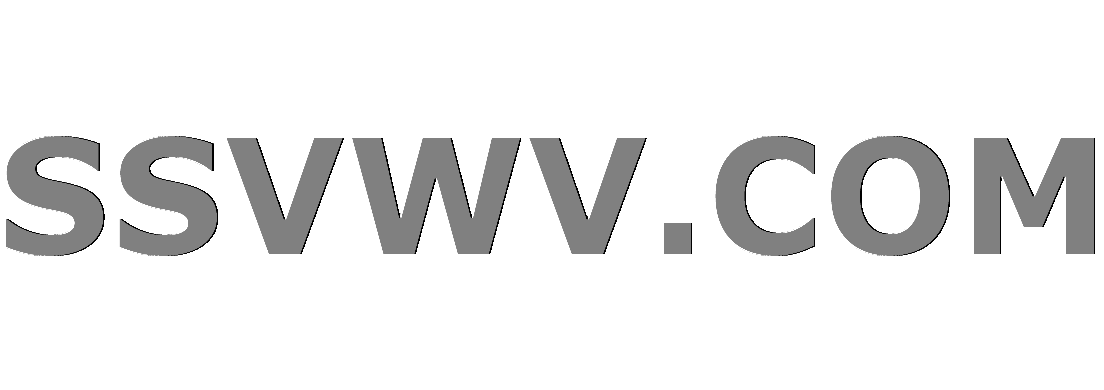
Multi tool use
If I want to read all integers from standard input to a vector, I can use the handy:
vector<int> v{istream_iterator<int>(cin), istream_iterator()};
But let's assume I only want to read n
integers. Is the hand-typed loop everything I got?
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
cin >> v[i];
Or is there any more right-handed way to do this?
c++ vector input
add a comment |
If I want to read all integers from standard input to a vector, I can use the handy:
vector<int> v{istream_iterator<int>(cin), istream_iterator()};
But let's assume I only want to read n
integers. Is the hand-typed loop everything I got?
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
cin >> v[i];
Or is there any more right-handed way to do this?
c++ vector input
1
Possible duplicate of std::copy n elements or to the end
– bartop
Jan 18 at 14:37
add a comment |
If I want to read all integers from standard input to a vector, I can use the handy:
vector<int> v{istream_iterator<int>(cin), istream_iterator()};
But let's assume I only want to read n
integers. Is the hand-typed loop everything I got?
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
cin >> v[i];
Or is there any more right-handed way to do this?
c++ vector input
If I want to read all integers from standard input to a vector, I can use the handy:
vector<int> v{istream_iterator<int>(cin), istream_iterator()};
But let's assume I only want to read n
integers. Is the hand-typed loop everything I got?
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
cin >> v[i];
Or is there any more right-handed way to do this?
c++ vector input
c++ vector input
edited Jan 18 at 14:04


JorgeAmVF
509520
509520
asked Jan 18 at 12:30
gaazkamgaazkam
2,163938
2,163938
1
Possible duplicate of std::copy n elements or to the end
– bartop
Jan 18 at 14:37
add a comment |
1
Possible duplicate of std::copy n elements or to the end
– bartop
Jan 18 at 14:37
1
1
Possible duplicate of std::copy n elements or to the end
– bartop
Jan 18 at 14:37
Possible duplicate of std::copy n elements or to the end
– bartop
Jan 18 at 14:37
add a comment |
2 Answers
2
active
oldest
votes
As given in comments, copy_n
is unsafe for this job, but you can use copy_if
with mutable lambda:
#include <iterator>
#include <vector>
#include <iostream>
#include <algorithm>
int main(){
const int N = 10;
std::vector<int> v;
//optionally v.reserve(N);
std::copy_if(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
std::back_inserter(v),
[count=N] (int) mutable {
return count && count--;
});
return 0;
}
as pointed out in this answer:
std::copy n elements or to the end
7
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
1
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
2
You can't fix it.std::copy_n
is not fit for this task.
– Lightness Races in Orbit
Jan 18 at 13:34
3
Still no. Dereferencing thestd::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)
– Lightness Races in Orbit
Jan 18 at 13:46
1
@bartop I suggest removing thecopy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.
– Ted Lyngmo
Jan 18 at 13:59
|
show 2 more comments
You usually shouldn't do this with std::copy_n
, which assumes that the provided iterator, when incremented n times, remains valid:
Copies exactly
count
values from the range beginning atfirst
to the range beginning atresult
. Formally, for each non-negative integeri < n
, performs*(result + i) = *(first + i)
.
(cppreference.com article on
std::copy_n
)
If you can guarantee that, then fine, but generally with std::cin
that's not possible. You can quite easily have it dereferencing an invalid iterator:
The default-constructed
std::istream_iterator
is known as the end-of-stream iterator. When a validstd::istream_iterator
reaches the end of the underlying stream, it becomes equal to the end-of-stream iterator. Dereferencing or incrementing it further invokes undefined behavior.
(cppreference.com article on
std::istream_iterator
)
You're pretty much there with your loop, though I'd probably use stronger termination condition to avoid excess reads from a "dead" stream:
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
if (!cin >> v[i])
break;
I'd be tempted actually to wrap this into something that's like std::copy_n
, but accepts a full "range" whose bounds may be validated in addition to counting from 0 to N.
An implementation might look like:
template<class InputIt, class Size, class OutputIt>
OutputIt copy_atmost_n(InputIt first, InputIt last, Size count, OutputIt result)
{
for (Size i = 0; i < count && first != last; ++i)
*result++ = *first++;
return result;
}
You'd use it like this:
copy_atmost_n(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
N,
std::back_inserter(v)
);
Now you get M elements, where M is either the number of inputs provided or N, whichever is smaller.
(live demo)
To confirm I’m interpreting this answer correctly, the issue withcopy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not usecopy_n
?”
– templatetypedef
Jan 18 at 16:37
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to usecopy_atmost_n
from hereon tbh
– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54254075%2fhow-to-read-n-integers-into-a-vector%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As given in comments, copy_n
is unsafe for this job, but you can use copy_if
with mutable lambda:
#include <iterator>
#include <vector>
#include <iostream>
#include <algorithm>
int main(){
const int N = 10;
std::vector<int> v;
//optionally v.reserve(N);
std::copy_if(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
std::back_inserter(v),
[count=N] (int) mutable {
return count && count--;
});
return 0;
}
as pointed out in this answer:
std::copy n elements or to the end
7
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
1
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
2
You can't fix it.std::copy_n
is not fit for this task.
– Lightness Races in Orbit
Jan 18 at 13:34
3
Still no. Dereferencing thestd::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)
– Lightness Races in Orbit
Jan 18 at 13:46
1
@bartop I suggest removing thecopy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.
– Ted Lyngmo
Jan 18 at 13:59
|
show 2 more comments
As given in comments, copy_n
is unsafe for this job, but you can use copy_if
with mutable lambda:
#include <iterator>
#include <vector>
#include <iostream>
#include <algorithm>
int main(){
const int N = 10;
std::vector<int> v;
//optionally v.reserve(N);
std::copy_if(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
std::back_inserter(v),
[count=N] (int) mutable {
return count && count--;
});
return 0;
}
as pointed out in this answer:
std::copy n elements or to the end
7
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
1
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
2
You can't fix it.std::copy_n
is not fit for this task.
– Lightness Races in Orbit
Jan 18 at 13:34
3
Still no. Dereferencing thestd::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)
– Lightness Races in Orbit
Jan 18 at 13:46
1
@bartop I suggest removing thecopy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.
– Ted Lyngmo
Jan 18 at 13:59
|
show 2 more comments
As given in comments, copy_n
is unsafe for this job, but you can use copy_if
with mutable lambda:
#include <iterator>
#include <vector>
#include <iostream>
#include <algorithm>
int main(){
const int N = 10;
std::vector<int> v;
//optionally v.reserve(N);
std::copy_if(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
std::back_inserter(v),
[count=N] (int) mutable {
return count && count--;
});
return 0;
}
as pointed out in this answer:
std::copy n elements or to the end
As given in comments, copy_n
is unsafe for this job, but you can use copy_if
with mutable lambda:
#include <iterator>
#include <vector>
#include <iostream>
#include <algorithm>
int main(){
const int N = 10;
std::vector<int> v;
//optionally v.reserve(N);
std::copy_if(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
std::back_inserter(v),
[count=N] (int) mutable {
return count && count--;
});
return 0;
}
as pointed out in this answer:
std::copy n elements or to the end
edited Jan 18 at 14:37
answered Jan 18 at 12:40


bartopbartop
2,855826
2,855826
7
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
1
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
2
You can't fix it.std::copy_n
is not fit for this task.
– Lightness Races in Orbit
Jan 18 at 13:34
3
Still no. Dereferencing thestd::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)
– Lightness Races in Orbit
Jan 18 at 13:46
1
@bartop I suggest removing thecopy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.
– Ted Lyngmo
Jan 18 at 13:59
|
show 2 more comments
7
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
1
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
2
You can't fix it.std::copy_n
is not fit for this task.
– Lightness Races in Orbit
Jan 18 at 13:34
3
Still no. Dereferencing thestd::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)
– Lightness Races in Orbit
Jan 18 at 13:46
1
@bartop I suggest removing thecopy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.
– Ted Lyngmo
Jan 18 at 13:59
7
7
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
Wouldn't it cause UB if there is not enough input provided?
– paler123
Jan 18 at 12:55
1
1
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
Indeed, this is incorrect.
– Lightness Races in Orbit
Jan 18 at 13:29
2
2
You can't fix it.
std::copy_n
is not fit for this task.– Lightness Races in Orbit
Jan 18 at 13:34
You can't fix it.
std::copy_n
is not fit for this task.– Lightness Races in Orbit
Jan 18 at 13:34
3
3
Still no. Dereferencing the
std::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)– Lightness Races in Orbit
Jan 18 at 13:46
Still no. Dereferencing the
std::istream_iterator
"end iterator" has UB, not throw semantics. (See my answer)– Lightness Races in Orbit
Jan 18 at 13:46
1
1
@bartop I suggest removing the
copy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.– Ted Lyngmo
Jan 18 at 13:59
@bartop I suggest removing the
copy_n
part and just leave As given in comments, copy_n is unsafe, but you can use copy_if with mutable lambda: followed by your nice solution.– Ted Lyngmo
Jan 18 at 13:59
|
show 2 more comments
You usually shouldn't do this with std::copy_n
, which assumes that the provided iterator, when incremented n times, remains valid:
Copies exactly
count
values from the range beginning atfirst
to the range beginning atresult
. Formally, for each non-negative integeri < n
, performs*(result + i) = *(first + i)
.
(cppreference.com article on
std::copy_n
)
If you can guarantee that, then fine, but generally with std::cin
that's not possible. You can quite easily have it dereferencing an invalid iterator:
The default-constructed
std::istream_iterator
is known as the end-of-stream iterator. When a validstd::istream_iterator
reaches the end of the underlying stream, it becomes equal to the end-of-stream iterator. Dereferencing or incrementing it further invokes undefined behavior.
(cppreference.com article on
std::istream_iterator
)
You're pretty much there with your loop, though I'd probably use stronger termination condition to avoid excess reads from a "dead" stream:
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
if (!cin >> v[i])
break;
I'd be tempted actually to wrap this into something that's like std::copy_n
, but accepts a full "range" whose bounds may be validated in addition to counting from 0 to N.
An implementation might look like:
template<class InputIt, class Size, class OutputIt>
OutputIt copy_atmost_n(InputIt first, InputIt last, Size count, OutputIt result)
{
for (Size i = 0; i < count && first != last; ++i)
*result++ = *first++;
return result;
}
You'd use it like this:
copy_atmost_n(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
N,
std::back_inserter(v)
);
Now you get M elements, where M is either the number of inputs provided or N, whichever is smaller.
(live demo)
To confirm I’m interpreting this answer correctly, the issue withcopy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not usecopy_n
?”
– templatetypedef
Jan 18 at 16:37
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to usecopy_atmost_n
from hereon tbh
– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
add a comment |
You usually shouldn't do this with std::copy_n
, which assumes that the provided iterator, when incremented n times, remains valid:
Copies exactly
count
values from the range beginning atfirst
to the range beginning atresult
. Formally, for each non-negative integeri < n
, performs*(result + i) = *(first + i)
.
(cppreference.com article on
std::copy_n
)
If you can guarantee that, then fine, but generally with std::cin
that's not possible. You can quite easily have it dereferencing an invalid iterator:
The default-constructed
std::istream_iterator
is known as the end-of-stream iterator. When a validstd::istream_iterator
reaches the end of the underlying stream, it becomes equal to the end-of-stream iterator. Dereferencing or incrementing it further invokes undefined behavior.
(cppreference.com article on
std::istream_iterator
)
You're pretty much there with your loop, though I'd probably use stronger termination condition to avoid excess reads from a "dead" stream:
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
if (!cin >> v[i])
break;
I'd be tempted actually to wrap this into something that's like std::copy_n
, but accepts a full "range" whose bounds may be validated in addition to counting from 0 to N.
An implementation might look like:
template<class InputIt, class Size, class OutputIt>
OutputIt copy_atmost_n(InputIt first, InputIt last, Size count, OutputIt result)
{
for (Size i = 0; i < count && first != last; ++i)
*result++ = *first++;
return result;
}
You'd use it like this:
copy_atmost_n(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
N,
std::back_inserter(v)
);
Now you get M elements, where M is either the number of inputs provided or N, whichever is smaller.
(live demo)
To confirm I’m interpreting this answer correctly, the issue withcopy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not usecopy_n
?”
– templatetypedef
Jan 18 at 16:37
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to usecopy_atmost_n
from hereon tbh
– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
add a comment |
You usually shouldn't do this with std::copy_n
, which assumes that the provided iterator, when incremented n times, remains valid:
Copies exactly
count
values from the range beginning atfirst
to the range beginning atresult
. Formally, for each non-negative integeri < n
, performs*(result + i) = *(first + i)
.
(cppreference.com article on
std::copy_n
)
If you can guarantee that, then fine, but generally with std::cin
that's not possible. You can quite easily have it dereferencing an invalid iterator:
The default-constructed
std::istream_iterator
is known as the end-of-stream iterator. When a validstd::istream_iterator
reaches the end of the underlying stream, it becomes equal to the end-of-stream iterator. Dereferencing or incrementing it further invokes undefined behavior.
(cppreference.com article on
std::istream_iterator
)
You're pretty much there with your loop, though I'd probably use stronger termination condition to avoid excess reads from a "dead" stream:
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
if (!cin >> v[i])
break;
I'd be tempted actually to wrap this into something that's like std::copy_n
, but accepts a full "range" whose bounds may be validated in addition to counting from 0 to N.
An implementation might look like:
template<class InputIt, class Size, class OutputIt>
OutputIt copy_atmost_n(InputIt first, InputIt last, Size count, OutputIt result)
{
for (Size i = 0; i < count && first != last; ++i)
*result++ = *first++;
return result;
}
You'd use it like this:
copy_atmost_n(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
N,
std::back_inserter(v)
);
Now you get M elements, where M is either the number of inputs provided or N, whichever is smaller.
(live demo)
You usually shouldn't do this with std::copy_n
, which assumes that the provided iterator, when incremented n times, remains valid:
Copies exactly
count
values from the range beginning atfirst
to the range beginning atresult
. Formally, for each non-negative integeri < n
, performs*(result + i) = *(first + i)
.
(cppreference.com article on
std::copy_n
)
If you can guarantee that, then fine, but generally with std::cin
that's not possible. You can quite easily have it dereferencing an invalid iterator:
The default-constructed
std::istream_iterator
is known as the end-of-stream iterator. When a validstd::istream_iterator
reaches the end of the underlying stream, it becomes equal to the end-of-stream iterator. Dereferencing or incrementing it further invokes undefined behavior.
(cppreference.com article on
std::istream_iterator
)
You're pretty much there with your loop, though I'd probably use stronger termination condition to avoid excess reads from a "dead" stream:
vector<int> v(n);
for(vector<int>::size_type i = 0; i < n; i++)
if (!cin >> v[i])
break;
I'd be tempted actually to wrap this into something that's like std::copy_n
, but accepts a full "range" whose bounds may be validated in addition to counting from 0 to N.
An implementation might look like:
template<class InputIt, class Size, class OutputIt>
OutputIt copy_atmost_n(InputIt first, InputIt last, Size count, OutputIt result)
{
for (Size i = 0; i < count && first != last; ++i)
*result++ = *first++;
return result;
}
You'd use it like this:
copy_atmost_n(
std::istream_iterator<int>(std::cin),
std::istream_iterator<int>(),
N,
std::back_inserter(v)
);
Now you get M elements, where M is either the number of inputs provided or N, whichever is smaller.
(live demo)
edited Jan 18 at 14:06
answered Jan 18 at 13:34


Lightness Races in OrbitLightness Races in Orbit
287k51466791
287k51466791
To confirm I’m interpreting this answer correctly, the issue withcopy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not usecopy_n
?”
– templatetypedef
Jan 18 at 16:37
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to usecopy_atmost_n
from hereon tbh
– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
add a comment |
To confirm I’m interpreting this answer correctly, the issue withcopy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not usecopy_n
?”
– templatetypedef
Jan 18 at 16:37
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to usecopy_atmost_n
from hereon tbh
– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
To confirm I’m interpreting this answer correctly, the issue with
copy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not use copy_n
?”– templatetypedef
Jan 18 at 16:37
To confirm I’m interpreting this answer correctly, the issue with
copy_n
is that if the stream encounters a problem before reading n elements, the behavior is undefined? So basically, “if you trust your data source, go for it, but if you don’t, do not use copy_n
?”– templatetypedef
Jan 18 at 16:37
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to use
copy_atmost_n
from hereon tbh– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Pretty much - and if you "go for it" you save an iterator comparison on each step I suppose. But for general usage I'm going to use
copy_atmost_n
from hereon tbh– Lightness Races in Orbit
Jan 18 at 16:54
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
@templatetypedef Note that I'm thinking of EOF specifically - tbh I'm not sure what happens if there's data but read/parse fails
– Lightness Races in Orbit
Jan 18 at 16:55
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f54254075%2fhow-to-read-n-integers-into-a-vector%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
dw78yvjMwu5l 2
1
Possible duplicate of std::copy n elements or to the end
– bartop
Jan 18 at 14:37